Connecting Wallets
The SDK supports 350+ wallets out of the box, all you need to pass is their id.
Using Components
You can use ConnectButton
or ConnectEmbed
component for a quick, easy and customizable UI.
These components provide a beautiful UI for connecting various wallets and take care of a lot of wallet-specific edge cases - so you can focus on building your app.
These components support over 300+ wallets, including support in-app wallets and account abstraction.
It also automatically shows all installed EIP-6963
compliant wallet extensions installed by the user.
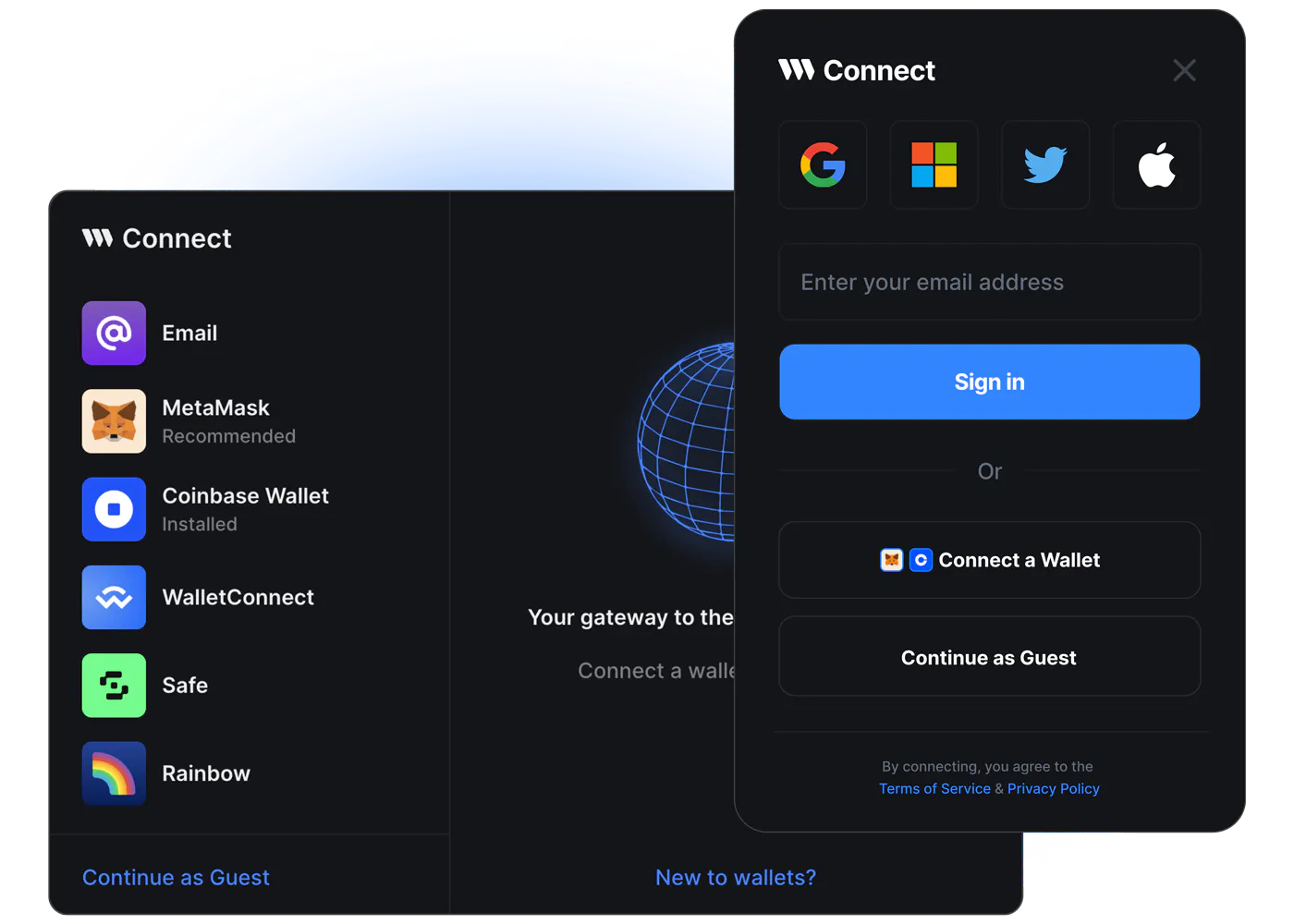
Using Hooks
You can build a completely custom UI for connecting the wallet using the useConnect
hook to connect any of support wallets.
Refer to createWallet
and injectedProvider
for more information.
Post Connection
Once the wallet is connected, you can use the Wallet Connection hooks to get information about the connected wallet like getting the address, account, etc